代码实现
- 这份代码不算是我写的吧.选课的实现也很有意思,student的节点有俩指针域,next指针用来维持链表,choice指针指向了选择的课程是最简单的,但是这样做一个学生选择两门课就会出现指针不够的问题,解决方法是让所选择的课程用next指出一个新的链表,但是next已经被course链表占用了,所以用了memcpy将course的节点拷贝出来,给每个学生节点选择的课程形成一张新的链表,然后插到student链表上,有点像树的数据结构了(还没学不清楚)
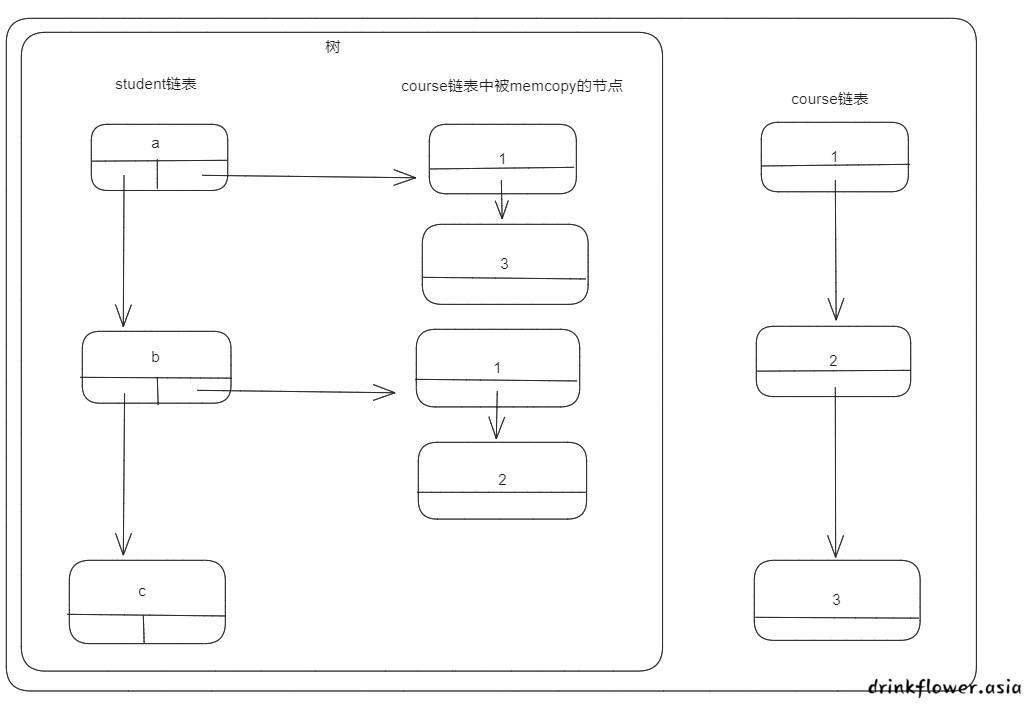
#include <stdio.h>
#include <stdlib.h>
#include <malloc.h>
#include <string.h>
#include <conio.h>
#include <unistd.h>
#include <stdbool.h>
#define MAX_PASSWORD_LENGTH 20
//尾插法
const char *filename = "list.txt";//写入文件名
int login=0;//验证教师权限
typedef struct Course{
char name[20]; //课程名称
int number; //课程序号
char teacher[20]; //课程教师姓名
int time; //课程课时
int classroom; //课程教室
struct Course *next; //链表next
}course;
typedef struct student{
int number; //学生序号
char name[20]; //学生姓名
char sex[20]; //学生性别
struct Course *choices; //学生选课
struct student *next; //链表next
}student;
//文件行数统计,用来确定需要创建链表的节点数
//int count_lines(const char* filename) {
// FILE* fp;
// int lines = 0;
// char ch;
//
// fp = fopen(filename, "r");
// if(fp == NULL) {
// printf("无法打开文件!\n");
// return -1;
// }
//
// while((ch = fgetc(fp)) != EOF) {
// if(ch == '\n') {
// lines++;
// }
// }
//
// fclose(fp);
// return lines;
//}
//写入到文件失败
//void save(student* student_head,course* course_head){
//FILE* fp = fopen("s.txt", "w");
// student* pow = student_head->next;
//
// while (pow) {
// course* pow2=pow->choices->next;
// while (pow2) {
// //printf("miao");
// fprintf(fp, "%s\t%s\t%s\t%d\t%s\t%d\t%d\n", pow->name, pow->sex, pow2->name, pow2->number, pow2->teacher,pow2->time,pow2->classroom);
// pow2 = pow2->next;
// }
// pow = pow->next;
// }
// fclose(fp);
//}
bool verifyPassword(const char *password) {
printf("请输入密码:\n");
char inputPassword[MAX_PASSWORD_LENGTH + 1];
int i = 0;
while (i < MAX_PASSWORD_LENGTH) {
inputPassword[i] = getch();
if (inputPassword[i] == '\r') {
inputPassword[i] = '\0';
break;
}
printf("*");
i++;
}
if (strcmp(inputPassword, password) == 0) {
return true;
} else {
return false;
}
}
//查找学生链表节点
student* find_student(student* student_head,int number){
if(student_head==NULL)return NULL;
if(student_head->number==number)return student_head;
return find_student(student_head->next, number);
}
//初始化链表
course* creat_course_start(course* course_head,char name[20],int number,char teacher[20],int time,int classroom){
course* new_course=(course*)malloc(sizeof(course));
strcpy(new_course->name,name);
new_course->number=number;
strcpy(new_course->teacher,teacher);
new_course->time=time;
new_course->classroom=classroom;
new_course->next=NULL;
return new_course;
}
//查找课程链表
course* find_course(course* course_head,int number){
if(course_head==NULL)return NULL;
if(course_head->number==number)return course_head;
return find_course(course_head->next, number); //使用递归进行搜索
}
//创建课程链表节点
course* creat_course(course* course_head){
course* new_course=(course*)malloc(sizeof(course));
printf("\n");
printf("\t\t--------- 输入课程信息 ------------\n");
printf("\n");
printf("请输入课程名称:");
scanf("%s",new_course->name);
printf("请输入课程序号:");
scanf("%d",&new_course->number);
printf("请输入教师姓名:");
scanf("%s",new_course->teacher);
printf("请输入课程课时:");
scanf("%d",&new_course->time);
printf("请输入课程教室:");
scanf("%d",&new_course->classroom);
while (find_course(course_head,new_course->number)!=NULL){
printf("此序号已经有数据,请重新输入.");
scanf("%d",&new_course->number);
}
new_course->next=NULL;
//读取文件行数,循环创建链表节点
// int n=count_lines(filename);
// for(int i=1;i<=n;i++){
//
// }
return new_course;
}
//头插法将新节点变成链表头部
course* add_course(course* course_head,course* new_course){
if(course_head==NULL)return new_course;
new_course->next=course_head;
return new_course;
}
//输出课程信息
void out_course(course* course_head){
while(course_head){
printf("\t%s\t\t%d\t\t%s\t\t%d\t\t%d\n",course_head->name,course_head->number,course_head->teacher,course_head->time,course_head->classroom);
course_head=course_head->next;
}
}
//删除课程信息
course* del_course(course* course_head,course* del_point){
course* point;
if(del_point == NULL){
printf("没有此序号信息,请重新输入!\n");
return course_head;
}
point=NULL;
if(del_point == course_head ){
point=course_head->next;
free(course_head);
return point;
}
point=course_head;
while(point){//搜索需要删除的节点
if(point->next == del_point){
point->next=del_point->next;
free(del_point);
return course_head;
}
point = point->next;
}
}
//创建链表,学生信息
student* creat_student(student* student_head){
student* new_student=(student*)malloc(sizeof(student));
printf("\n");
printf("\t\t--------- 输入学生信息 ------------\n");
printf("\n");
printf("请输入学生姓名:");
scanf("%s",new_student->name);
printf("请输入学生学号:");
scanf("%d",&new_student->number);
printf("请输入学生性别:");
scanf("%s",new_student->sex);
while (find_student(student_head,new_student->number)!=NULL){
printf("此学号已经有数据,请重新输入.");
scanf("%d",&new_student->number);
}
new_student->choices=NULL;
new_student->next=NULL;
return new_student;
}
//初始化学生链表
student* creat_student_start(student* student_head,char name[20],int number,char sex[20]){
student* new_student=(student*)malloc(sizeof(student));
strcpy(new_student->name,name);
new_student->number=number;
strcpy(new_student->sex,sex);
new_student->choices=NULL;
new_student->next=NULL;
return new_student;
}
//学生信息加入链表
student* add_student(student* student_head,student* new_student){
if(student_head==NULL)return new_student;
new_student->next=student_head;
return new_student;
}
//输出学生信息
void out_student(student* student_head){
while(student_head){
printf("\t%s\t\t%d\t\t%s\n",student_head->name,student_head->number,student_head->sex);
student_head=student_head->next;
}
}
//删除学生信息
student* del_student(student* student_head,student* del_point){
student* point;
if(del_point == NULL){
printf("没有此学号信息,请重新输入!\n");
return student_head;
}
point=NULL;
if(del_point == student_head ){
point=student_head->next;
free(student_head);
return point;
}
point=student_head;
while(point){
if(point->next == del_point){
point->next=del_point->next;
free(del_point);
return student_head;
}
point = point->next;
}
}
//学生选课
student* choice_course(student* student_head,int number1,course* course_head){
int number;
course* point=NULL;
course* choice_point=NULL;
student* student_point=find_student(student_head,number1);
if(student_point!=NULL){
printf("\t课程名称\t课程序号\t教师姓名\t课程课时\t课程教室\n");
out_course(course_head);
printf("请输入所选课程序号:");
scanf("%d",&number);
point=find_course(course_head,number);
if(point!=NULL){
choice_point=(course*)malloc(sizeof(course));
memcpy(choice_point,point,sizeof(course));
choice_point->next=NULL;
if(student_point->choices==NULL){
student_point->choices=choice_point;
}
else {
choice_point->next=student_point->choices;
student_point->choices=choice_point;
}
printf("恭喜你!选课成功!\n");
//save(student_head,course_head);
return student_head;
}
else{
printf("没有所选课程序号!");
return student_head;
}
}
else{
printf("没有所选学生学号!");
return student_head;
}
}
//输出学生选课信息
void out_choice(student* student_head){
course* point=NULL;
while(student_head){
point=student_head->choices;
while(point){
printf("\t%s\t\t%d\t\t%s",student_head->name,student_head->number,student_head->sex);
printf("\t\t%s\t\t%d\t\t%s\t\t%d\t\t%d\n",point->name,point->number,point->teacher,point->time,point->classroom);
point=point->next;
}
if(!point)printf("\n");
student_head=student_head->next;
}
}
void start(){
printf("\t--------- 1.录入课程 ------------\n");
printf("\t--------- 2.浏览课程 ------------\n");
printf("\t--------- 3.删除课程 ------------\n");
printf("\t--------- 4.输入学生信息 ------------\n");
printf("\t--------- 5.浏览学生信息 ------------\n");
printf("\t--------- 6.删除学生信息 ------------\n");
printf("\t--------- 7.学生选课 ------------\n");
printf("\t--------- 8.所有学生选课信息 ------------\n");
printf("\t--------- 9.教师登录 ------------\n");
printf("\t--------- 10.退出程序 ------------\n");
}
int main(){
int a,n=0,i;
int number=0;
int number1=0;
course* course_head=NULL;
student* student_head=NULL;
course* new_course=NULL;
student* new_student=NULL;
course* del_point=NULL;
char choice[20];
//读入初始化数据
new_course=creat_course_start(course_head,"工程实践",1,"蜂考",16,4338);
course_head=add_course(course_head,new_course);
new_course=creat_course_start(course_head,"Java程序设计",2,"百度",22,3028);
course_head=add_course(course_head,new_course);
new_course=creat_course_start(course_head,"离散数学",3,"必应",23,2222);
course_head=add_course(course_head,new_course);
new_course=creat_course_start(course_head,"线性代数",4,"谷歌",24,3002);
course_head=add_course(course_head,new_course);
new_course=creat_course_start(course_head,"高等数学",5,"坤坤",18,3028);
course_head=add_course(course_head,new_course);
new_student=creat_student_start(student_head,"坤坤",0,"男");
student_head=add_student(student_head,new_student);
new_student=creat_student_start(student_head,"羊宝",1,"男");
student_head=add_student(student_head,new_student);
new_student=creat_student_start(student_head,"狐狸",2,"女");
student_head=add_student(student_head,new_student);
new_student=creat_student_start(student_head,"鱼头",3,"男");
student_head=add_student(student_head,new_student);
new_student=creat_student_start(student_head,"十三",4,"女");
student_head=add_student(student_head,new_student);
do{
system("cls");
start();
printf("请在1-10中选择:");
scanf("%d",&a);
switch(a){
case 1:
system("cls");
if(login==0){
printf("请先进行教师登录!");
sleep(2);
break;
}
new_course=creat_course(course_head);
course_head=add_course(course_head,new_course);
//save_course_to_file(course_head, filename);
break;
case 2:
system("cls");
printf("\t\t--------- 全部课程信息 ------------\n");
printf("\t课程名称\t课程序号\t教师姓名\t课程课时\t课程教室\n");
out_course(course_head);
system("pause");
break;
case 3:
system("cls");
if(login==0){
printf("请先进行教师登录!");
sleep(2);
break;
}
printf("\t课程名称\t课程序号\t教师姓名\t课程课时\t课程教室\n");
out_course(course_head);
printf("请输入需要删除的课程序号:\n");
scanf("%d",&number);
course_head=del_course(course_head,find_course(course_head,number));
printf("完成!\n");
system("pause");
break;
case 4:
system("cls");
if(login==0){
printf("请先进行教师登录!");
sleep(2);
break;
}
new_student=creat_student(student_head);
student_head=add_student(student_head,new_student);
break;
case 5:
system("cls");
printf("\t\t--------- 全部学生信息 ------------\n");
printf("\t学生姓名\t学生学号\t学生性别\n");
out_student(student_head);
system("pause");
break;
case 6:
system("cls");
if(login==0){
printf("请先进行教师登录!");
sleep(2);
break;
}
printf("请输入需要删除的学生的学号:\n");
scanf("%d",&number);
student_head=del_student(student_head,find_student(student_head,number));
printf("完成!\n");
system("pause");
break;
case 7:
system("cls");
printf("\t学生姓名\t学生学号\t学生性别\n");
out_student(student_head);
printf("请输入选课学生的学号:");
scanf("%d",&number1);
choice_course(student_head,number1,course_head);
//save_course_to_file(student_head,filename);
system("pause");
break;
case 8:
system("cls");
printf("\t\t\t\t--------- 全部选课信息 ------------\n");
printf("\n");
printf("\t学生姓名\t学生学号\t学生性别\t课程名称\t课程序号\t教师姓名\t课程课时\t课程教室\n");
out_choice(student_head);
system("pause");
break;
case 9:
system("cls");
//printf("请输入密码\n");
if(verifyPassword("administractor")==true){
login=1;
printf("密码正确!即将返回");
sleep(2);
}
else{
printf("密码错误!即将返回");
sleep(2);
}
break;
case 10:
return 0;
break;
}
} while(a!=0);
return 0;
}
文件读存
- 写入和读取文件的功能暂时没有整合进去,但是代码是有的
#define _CRT_SECURE_NO_WARNINGS
////
#include <stdio.h>
#include<stdlib.h>
#include<String.h>
//定义结构题和声明函数
struct Student {
char number[20];
char name[20];
char teacher[20];
char majoy[20];
};
struct Node {
struct Student data;
struct Node2* next2;
struct Node* next;
};
struct Course {
char name[30];
};
struct Node2 {//课程节点
struct Course data2;
struct Node2* next;
};
struct Node* GreatNode() {
struct Node* HeadNode = (struct Node*)malloc(sizeof(struct Node));
HeadNode->next = NULL;
return HeadNode;
}
struct Node* GreatNewNode(struct Student data) {
struct Node* NewNode = (struct Node*)malloc(sizeof(struct Node2));
NewNode->data = data;
NewNode->next = NULL;
return NewNode;
}
void GreadNodeList(struct Node* HeadNode, struct Student data) {
struct Node* NewNode = GreatNewNode(data);
NewNode->next = HeadNode->next;
HeadNode->next=NewNode;
}
/// /////////////////////////////////////////////////////////////////////////////////////////////////////////////
struct Node2* GreadNode2() {
struct Node2* HeadNode = (struct Node2*)malloc(sizeof(struct Node2));
HeadNode->next = NULL;
return HeadNode;
}
struct Node2* GreadNewNode2(struct Course data) {
struct Node2* NewNode = (struct Node2*)malloc(sizeof(struct Node2));
NewNode->data2 = data;
NewNode->next = NULL;
return NewNode;
}
void GreadNodeList2(struct Node2* HeadNode, struct Course data) {
struct Node2* NewNode = GreadNewNode2(data);
NewNode->next = HeadNode->next;
HeadNode->next = NewNode;
}
struct Node* HeadNode;
int main() {
HeadNode = GreatNode();
int n;
printf("请输入你要输入的学生个数:");
scanf("%d", &n);
for (int i = 0; i < n; i++) {
struct Student data;
printf("请输入学生的学号:");
scanf("%s", &data.number);
printf("请输入学生的姓名:");
scanf("%s", &data.name);
printf("请输入学生的老师:");
scanf("%s", &data.teacher);
printf("请输入学生的专业:");
scanf("%s", &data.majoy);
GreadNodeList(HeadNode,data);
HeadNode->next->next2 = GreadNode2();
int x;//选课的数量
printf("请输入学生选课的数量:");
scanf("%d", &x);
for (int i = 0; i < x; i++) {
struct Course data2;
printf("请输入学生选课的名称:");
scanf("%s", &data2.name);
GreadNodeList2(HeadNode->next->next2, data2);
}
}
FILE* fp = fopen("s.txt", "w");
struct Node* pow = HeadNode->next;
while (pow) {
struct Node2* pow2=pow->next2->next;
while (pow2) {
fprintf(fp, "%s\t%s\t%s\t%s\t%s\n", pow->data.number, pow->data.name, pow->data.teacher, pow->data.majoy, pow2->data2.name);
pow2 = pow2->next;
}
pow = pow->next;
}
fclose(fp);
return 0;
}